Welcome to the new Parasoft forums! We hope you will enjoy the site and try out some of the new features, like sharing an idea you may have for one of our products or following a category.
User Defined Load Testing Stop Actions
Options
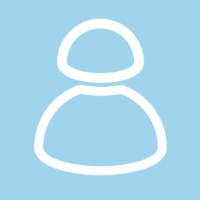
LegacyForum
Posts: 1,664 ✭✭
Sample Scripts
As of SOAPtest version 3.0.1 (Build date: November 22, 2004), user defined scripted Load Tester stop actions have been introduced. These stop actions allow you to stop the load tester from executing if a particular condition or state is reached. Some sample scripts that can be used are shown below:CODE
from java.lang import *
from java.util import *
from com.parasoft.api.loadtest import *
from com.parasoft.api.loadtest.output import *
#
# Print to console available merged data
#
def printMergedData(args):
print '************************'
resultSet = args.getOutput().getMergedOutput()
resultSet.resetRowIterator()
while resultSet.hasNextRow():
row = resultSet.nextRow()
entries = row.entrySet()
iter = entries.iterator()
print ' -------------'
while iter.hasNext():
entry = iter.next()
print ' ', entry.getKey(), entry.getValue()
#
# Print to console available curent data
#
def printCurrData(args):
print '************************'
iter = args.getOutput().getCurrentOutput().entrySet().iterator()
while iter.hasNext():
entry = iter.next()
print ' ', entry.getKey(), entry.getValue()
#
# Stop if total number of errors exceeds N
#
def stopAfterNErrors(args):
ERROR_THRESHOLD = 200
STOP_DESC = 'Number of errors exceeded 200.'
output = args.getOutput()
resultSet = output.getMergedOutput()
resultSet.resetRowIterator()
failures = 0
while resultSet.hasNextRow():
row = resultSet.nextRow()
failures += row.get(MergedOutputConstants.FAILURE_COUNT)
if failures > ERROR_THRESHOLD:
return LoadTestScriptAction(LoadTestScriptAction.ACTION_STOP,STOP_DESC)
#
# Stop if parameter N is above threshold T for M consecutive measurements
#
def stopWhenCPUHigh(args,context):
#-- Stop if CPU utilization is greater than 10% for 5 consecutive invocations
#-- Modify theese numbers according to your needs:
PARAM_THRESHOLD = 10
CONSECUTIVE_LIMIT = 5
PARAM_NAME = 'cheetah/CPU'
STOP_DESC = 'CPU utilization greater than 10% for 5 consecutive invocations'
#-- Store the consecutive count in context between invocations
contextValueID = 'COUNT'
count = context.get(contextValueID)
if count is None:
count = 0
context.put(contextValueID,count)
paramValue = args.getOutput().getCurrentOutput().get(PARAM_NAME)
print PARAM_NAME,'=',paramValue
if paramValue > PARAM_THRESHOLD:
count = count + 1
else:
count = 0
context.put(contextValueID,count)
if count >= CONSECUTIVE_LIMIT:
return LoadTestScriptAction(LoadTestScriptAction.ACTION_STOP,STOP_DESC)
#
# Do nothing
#
def doNothing(allData):
return 0
0
Comments
-
Hi,
When defining the following code in "Scenario -> Load Test stop action", what is the print statement printing to? There is no output in the console window, or the log. This is just a simple HTTP client accessing http://www.google.com [GET].CODEfrom java.lang import *
from java.util import *
from com.parasoft.api.loadtest import *
from com.parasoft.api.loadtest.output import *
# Print to console available curent data
#
def printCurrData(args):
print '************************'
iter = args.getOutput().getCurrentOutput().entrySet().iterator()
while iter.hasNext():
entry = iter.next()
print ' ', entry.getKey(), entry.getValue()0