GraphQL SDL tips
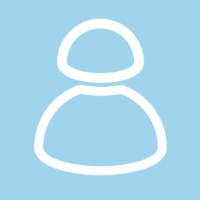
The SOAtest GraphQL Client has a Form GraphQL view that can be constrained to the fields defined in a GraphQL Schema Definition Language (SDL) document. This simplifies building queries, where the Form GraphQL view is populated with controls to select and configure the available fields, arguments, etc.
A GraphQL SDL may be available in the documentation or source repository of the GraphQL endpoint. Some public examples:
https://raw.githubusercontent.com/graphql/swapi-graphql/master/schema.graphql
https://docs.github.com/public/schema.docs.graphql
What if you do not have access to the GraphQL SDL document? What if it is difficult to obtain? If the GraphQL endpoint has introspection enabled then you can generate a GraphQL SDL document from an introspection result.
First, create a GraphQL Client. On the Resource tab, provide the URL of the GraphQL endpoint. On the Payload tab, configure a GraphQL introspection query:
query IntrospectionQuery { __schema { queryType { name } mutationType { name } subscriptionType { name } types { ...FullType } directives { name description locations args { ...InputValue } } } } fragment FullType on __Type { kind name description fields { name description args { ...InputValue } type { ...TypeRef } } inputFields { ...InputValue } interfaces { ...TypeRef } enumValues { name description } possibleTypes { ...TypeRef } } fragment InputValue on __InputValue { name description type { ...TypeRef } defaultValue } fragment TypeRef on __Type { kind name ofType { kind name ofType { kind name ofType { kind name ofType { kind name ofType { kind name ofType { kind name ofType { kind name } } } } } } } }
Next, chain an Extension Tool to the GraphQL Client's "Response Traffic" output. Use a script to convert the introspection result:
import com.fasterxml.jackson.core.type.* import com.fasterxml.jackson.databind.* import graphql.introspection.* import graphql.schema.idl.* String convertToSDL(def resultJson, def context) { def mapper = new ObjectMapper() def typeRef = new TypeReference<HashMap<String,Object>>() {} def introspectionResult = mapper.readValue(resultJson, typeRef).get("data") IntrospectionResultToSchema introspectionResultToSchema = new IntrospectionResultToSchema() def schemaIDL = introspectionResultToSchema.createSchemaDefinition(introspectionResult) def schemaPrinter = new SchemaPrinter() def sdlString = schemaPrinter.print(schemaIDL) return sdlString }
Lastly, chain a Write File tool to the Extension Tool's "Return Value" output. In the "Target name" field, type a file name for your GraphQL SDL document (MySDL.graphqls). In the "Target directory" field, type "." to save the file in the same directory as your SOAtest project.
Now, you have a GraphQL SDL document which you can use to build your queries in the Form GraphQL view.
Comments
-
GraphQL SDL can also be used for service virtualization.
See GraphQL SDL tips on the Virtualize forum for detail.
0