How to properly set up a unit test with a data source?
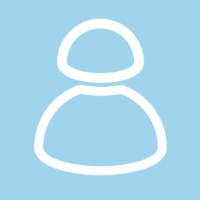
I'm trying to set up a unit test that imports a file of inputs and outputs as a data source. I think i've got the columns mapped properly, but every line reports "Signal SIGSEGV caught: segmentation fault [CPPTEST_SIGSEGV]", coming from the suite .cpp file where the data source is declared:
CPPTEST_TEST_DS(data_source_test_case, CPPTEST_DC("excel_data_source"));
I've recreated the test with a .xlsx and a .csv, and even copied a few lines into a custom table to see if there was a bad line ending in the files. But each time, every line fails with a segfault.
Am I missing something on how to create these tests?
PS. I am creating them in the editor, not the wizard.
Answers
-
I am writing down all the things related to unit test with a data source -
Our first attempt at a design for the MyBank application includes an accounts component that represents an individual account and its transactions with the bank, and a database component that represents the functionality to aggregate and manage the individual accounts.We create a MyBank solution that contains two projects:
Accounts
BankDb
Our first attempt at designing the Accounts project contains a class to hold basic information about an account, an interface that specifies the common functionality of any type of account, like depositing and withdrawing assets from the account, and a class derived from the interface that represents a checking account. We begin the Accounts projects by creating the following source files:
AccountInfo.cs defines the basic information for an account.
IAccount.cs defines a standard IAccount interface for an account, including methods to deposit and withdraw assets from an account and to retrieve the account balance.
CheckingAccount.cs contains the CheckingAccount class that implements the IAccount interface for a checking account.
We know from experience that one thing a withdrawal from a checking account must do is to make sure that the amount withdrawn is less than the account balance. So we override the IAccount.Withdraw method in CheckingAccount with a method that checks for this condition. The method might look like this:
C#
Copy
public void Withdraw(double amount)
{
if(m_balance >= amount)
{
m_balance -= amount;
}
else
{
throw new ArgumentException(nameof(amount), "Withdrawal exceeds balance!");
}
}
Now that we have some code, it's time for testing.Create unit test projects and test methods (C#)
For C#, it is often quicker to generate the unit test project and unit test stubs from your code. Or you can choose to create the unit test project and tests manually depending on your requirements. If you want to create unit tests from code with a 3rd party framework you will need one of these extensions installed: NUnit or xUnit. If you are not using C#, skip this section and go to Create the unit test project and unit tests manually.Generate unit test project and unit test stubs
From the code editor window, right-click and choose Create Unit Tests from the right-click menu.
Click OK to accept the defaults to create your unit tests, or change the values used to create and name the unit test project and the unit tests. You can select the code that is added by default to the unit test methods.
Create the unit test project and unit tests manually
A unit test project usually mirrors the structure of a single code project. In the MyBank example, you add two unit test projects named AccountsTests and BankDbTests to the MyBanks solution. The test project names are arbitrary, but adopting a standard naming convention is a good idea.To add a unit test project to a solution:
In Solution Explorer, right-click on the solution and choose Add > New Project.
Type test in the project template search box to find a unit test project template for the test framework that you want to use. (In the examples in this topic, we use MSTest.)On the next page, name the project. To test the Accounts project of our example, you could name the project AccountsTests.
In your unit test project, add a reference to the code project under test, in our example to the Accounts project.
To create the reference to the code project:
In the unit test project in Solution Explorer, right-click the References or Dependencies node, and then choose Add Project Reference or Add Reference, whichever is available.
On the Reference Manager dialog box, open the Solution node and choose Projects. Select the code project name and close the dialog box.
Each unit test project contains classes that mirror the names of the classes in the code project. In our example, the AccountsTests project would contain the following classes:
AccountInfoTests class contains the unit test methods for the AccountInfo class in the Accounts project
CheckingAccountTests class contains the unit test methods for CheckingAccount class.
Write your tests
The unit test framework that you use and Visual Studio IntelliSense will guide you through writing the code for your unit tests for a code project. To run in Test Explorer, most frameworks require that you add specific attributes to identify unit test methods. The frameworks also provide a way—usually through assert statements or method attributes—to indicate whether the test method has passed or failed. Basically, I have written a project fully based on the unit test method which you can check [best hrms software in kolkata](SANITIZED: https___www.exactlly.com_blog_index.php_gaining-insight-into-key-features-of-hrms-software_) and related things. Other attributes identify optional setup methods that are at class initialization and before each test method and teardown methods that are run after each test method and before the class is destroyed.The AAA (Arrange, Act, Assert) pattern is a common way of writing unit tests for a method under test.
The Arrange section of a unit test method initializes objects and sets the value of the data that is passed to the method under test.
The Act section invokes the method under test with the arranged parameters.
The Assert section verifies that the action of the method under test behaves as expected. For .NET, methods in the Assert class are often used for verification.
To test the CheckingAccount.Withdraw method of our example, we can write two tests: one that verifies the standard behavior of the method, and one that verifies that a withdrawal of more than the balance will fail (The following code shows an MSTest unit test, which is supported in .NET.). In the CheckingAccountTests class, we add the following methods:
C#
Copy
[TestMethod]
public void Withdraw_ValidAmount_ChangesBalance()
{
// arrange
double currentBalance = 10.0;
double withdrawal = 1.0;
double expected = 9.0;
var account = new CheckingAccount("JohnDoe", currentBalance);// act account.Withdraw(withdrawal); // assert Assert.AreEqual(expected, account.Balance);
}
[TestMethod]
public void Withdraw_AmountMoreThanBalance_Throws()
{
// arrange
var account = new CheckingAccount("John Doe", 10.0);// act and assert Assert.ThrowsException<System.ArgumentException>(() => account.Withdraw(20.0));
}
For more information about the Microsoft unit testing frameworks, see one of the following topics:Unit test your code
Writing unit tests for C/C++
Use the MSTest framework in unit tests
Set timeouts for unit tests
If you're using the MSTest framework, you can use the TimeoutAttribute to set a timeout on an individual test method:C#
Copy
[TestMethod]
[Timeout(2000)] // Milliseconds
public void My_Test()
{ ...
}
To set the timeout to the maximum allowed:C#
Copy
[TestMethod]
[Timeout(TestTimeout.Infinite)] // Milliseconds
public void My_Test ()
{ ...
}
Run tests in Test Explorer
When you build the test project, the tests appear in Test Explorer. If Test Explorer is not visible, choose Test on the Visual Studio menu, choose Windows, and then choose Test Explorer (or press Ctrl + E, T).As you run, write, and rerun your tests, the Test Explorer can display the results in groups of Failed Tests, Passed Tests, Skipped Tests and Not Run Tests. You can choose different group by options in the toolbar.
You can also filter the tests in any view by matching text in the search box at the global level or by selecting one of the pre-defined filters. You can run any selection of tests at any time. The results of a test run are immediately apparent in the pass/fail bar at the top of the explorer window. Details of a test method result are displayed when you select the test.
Run and view tests
The Test Explorer toolbar helps you discover, organize, and run the tests that you are interested in.
You can choose Run All to run all your tests (or press Ctrl + R, V), or choose Run to choose a subset of tests to run (Ctrl + R, T). Select a test to view the details of that test in the test details page. Choose Open Test from the right-click menu (Keyboard: F12) to display the source code for the selected test.If individual tests have no dependencies that prevent them from being run in any order, turn on parallel test execution in the settings menu of the toolbar. This can noticeably reduce the time taken to run all the tests.
Run tests after every build
To run your unit tests after each local build, open the settings icon in the Test Explorer toolbar and select Run Tests After Build.Filter and group the test list
When you have a large number of tests, you can type in the Test Explorer search box to filter the list by the specified string. You can restrict your filter even more by choosing from the filter list.
To create a data-driven test for the AddIntegerHelper method, we first create an Access database named AccountsTest.accdb and a table named AddIntegerHelperData. The AddIntegerHelperData table defines columns to specify the first and second operands of the addition and a column to specify the expected result. We fill a number of rows with appropriate values.C#
Copy
[DataSource(
@Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\Projects\MyBank\TestData\AccountsTest.accdb,
"AddIntegerHelperData"
)]
[TestMethod()]
public void AddIntegerHelper_DataDrivenValues_AllShouldPass()
{
var target = new CheckingAccount();
int x = Convert.ToInt32(TestContext.DataRow["FirstNumber"]);
int y = Convert.ToInt32(TestContext.DataRow["SecondNumber"]);
int expected = Convert.ToInt32(TestContext.DataRow["Sum"]);
int actual = target.AddIntegerHelper(x, y);
Assert.AreEqual(expected, actual);
}
The attributed method runs once for each row in the table. Test Explorer reports a test failure for the method if any of the iterations fail. The test results detail pane for the method shows you the pass/fail status method for each row of data.Learn more about data-driven unit tests.
Q: Can I view how much of my code is tested by my unit tests?
A: Yes. You can determine the amount of your code that is actually being tested by your unit tests by using the Visual Studio code coverage tool in Visual Studio Enterprise. Native and managed languages and all unit test frameworks that can be run by the Unit Test Framework are supported.
You can run code coverage on selected tests or on all tests in a solution. The Code Coverage Results window displays the percentage of the blocks of product code that were exercised by line, function, class, namespace and module.
To run code coverage for test methods in a solution, choose Test > Analyze Code Coverage for All Tests.
Hope this solution will help you properly.
-4 -
While I appreciate the time and effort you put into that response, I have a C++ project imported and have created test suites and unit tests already. The only place I'm running into trouble is when I try to run a test with a data source.
I'm looking for help setting up a data source unit test, for C/C++, either in the wizard or editor so that I do not get segfaults when I attempt to run it.
0