Welcome to the new Parasoft forums! We hope you will enjoy the site and try out some of the new features, like sharing an idea you may have for one of our products or following a category.
Compiling and running a JUnit test case
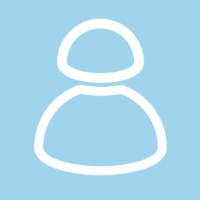
LegacyForum
Posts: 1,664 ✭✭
in FAQ
From the Windows command line
First, you can generate a JUnit Java file for a test scenario by selecting the test scenario in WebKing and choosing Tools > Generate Unit Test Cases. (You can also generate a JUnit Java file when recording a test scenario.)To compile and run the JUnit class using Java, your classpath will need to reference all of the JAR files in the WebKing installation directory. The Windows scripts junitcompile.bat and junitrun.bat (see below for the source code) automatically set Java's classpath.
For example, to compile and run MyScenario.java:
C:\somewhere>junitcompile.bat MyScenario.java
C:\somewhere>junitrun.bat MyScenario
You may need to modify the batch files to point to your WebKing installation and the Java SDK you want to use. Remove the comment indicator "rem" from the "rem echo %CLASSPATH%" line if you want to see the classpath that the script has generated.
junitcompile.bat:
CODE
@echo off
SETLOCAL ENABLEDELAYEDEXPANSION
SET WK="C:\Program Files\Parasoft\WebKing\6.0"
SET JAVA=C:\j2sdk1.4.2_18\bin\javac
SET CLASSPATH=.
for %%I in (%WK%\*.jar) do (
set CLASSPATH=!CLASSPATH!;"%%I")
rem echo %CLASSPATH%
%JAVA% -classpath %CLASSPATH% %1 %2 %3 %4 %5 %6 %7 %8 %9
junitrun.bat:
CODE@echo off
SETLOCAL ENABLEDELAYEDEXPANSION
SET WK="C:\Program Files\Parasoft\WebKing\6.0"
SET JAVA=C:\j2sdk1.4.2_18\bin\java
SET CLASSPATH=.
for %%I in (%WK%\*.jar) do (
set CLASSPATH=!CLASSPATH!;"%%I")
rem echo %CLASSPATH%
%JAVA% -classpath %CLASSPATH% %1 %2 %3 %4 %5 %6 %7 %8 %9
0
Comments
-
If you've upgraded to SOAtest 6.x, you can use the following instead to compile any Java code that utilizes the SOAtest API, such as a JUnit class or a class that is referenced from a Method Tool. The script makes sure to use any classes that have been updated in a service pack. Configure the parameters appropriately.CODE
@echo off
SETLOCAL ENABLEDELAYEDEXPANSION
set SOATEST_VERSION=6.1
set SOATEST_SERVICEPACK=1
set SOATEST_DIR=C:\Program Files (x86)\Parasoft\SOAtest\%SOATEST_VERSION%
set JAVA=C:\Program Files (x86)\Java\jdk1.5.0_18\bin\javac.exe
set CLASSPATH=.
rem Add service pack jars first, so they are first on the classpath.
set JAR_DIR=%SOATEST_DIR%\plugins\com.parasoft.xtest.libs.web.sp_%SOATEST_VERSION%.%SOATEST_SERVICEPACK%\root
for %%I in ("%JAR_DIR%"\*.jar) do (
set CLASSPATH=!CLASSPATH!;"%%I")
rem Add other SOAtest jars used for the scripting API.
rem Mount the directory with the jars as a drive letter, as otherwise the
rem CLASSPATH variable is longer than cmd.exe can handle.
subst /D z:
subst z: "%SOATEST_DIR%\plugins\com.parasoft.xtest.libs.web_%SOATEST_VERSION%.0\root"
set JAR_DIR=z:
for %%I in ("%JAR_DIR%"\*.jar) do (
set CLASSPATH=!CLASSPATH!;"%%I")
rem echo %CLASSPATH%
"%JAVA%" -classpath %CLASSPATH% %1 %2 %3 %4 %5 %6 %7 %8 %9
SOAtest does not ship with a JDK (which includes javac.exe), so you will need to use your own.
To run a Java class that uses the SOAtest API, change the JAVA variable to point to java.exe. You can use either your own java.exe or the one in the JRE that ships with SOAtest: [install dir]\plugins\com.parasoft.xtest.jre.eclipse.core.win32_xxx\jre0